Story Lab #
In this lab, you are introduced to the structure for writing a branching story. You will use this in your unit project.
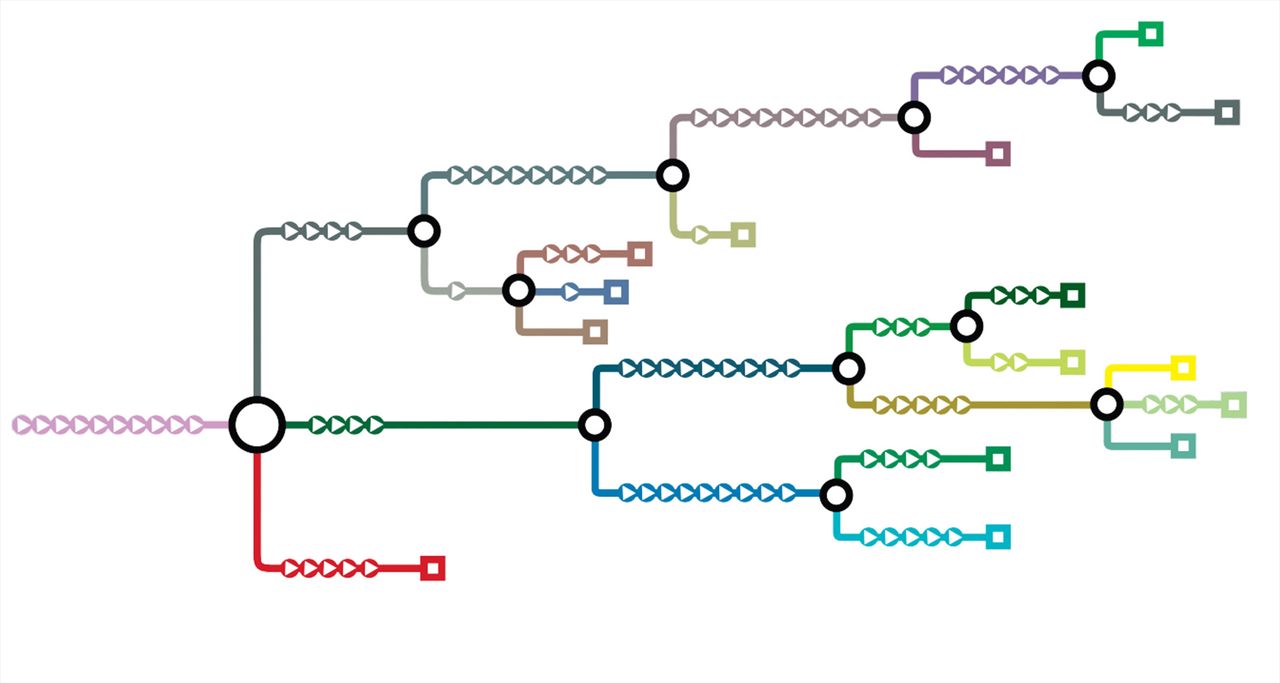
[0] Setup #
๐ Github Repo: github.com/Making-with-Code/lab_story
๐ป Enter the Poetry shell and install the requirements:poetry shell
poetry install
This repo includes the following files:
game.py
story_setup.py
view.py
node.py
story.py
[2] How do you write a story? #
Stories are made up of connected connected Node()
objects.
[Node] #
๐
Let’s start by looking at the Node
constructor:
class Node():
def __init__(self, id, option_title, description):
self.id = id
self.children = []
self.option_title = option_title
self.description = description
id
: a unique id (str
,float
, orinteger
)children
: a list ofNode()
objectsoption_title
: the text for the display in the menu (str
)description
: the additional text for when a user selects an option (str
)
It has the following methods:
__str__
: defines how aNode
is printedadd_child(child_node)
: this addschild_node
to itschildren
- this is how the story pieces are connected to each other.
find(id)
- searches through the nodes to return the node with theid
[Story] #
Now, let’s look at the Story()
constructor. This is the primary class you will be interacting with when writing your story.
class Story():
def __init__(self, title, start_id, start_option_title, start_description):
self.title = title
self.current_node = Node(start_id, start_option_title, start_description)
self.first_node = self.current_node
title
: the title of your story (`str)current_node
: the currentNode
of your story, at the start of the story it is the same asfirst_node
first_node
: the firstNode
of your story
A Story()
has 4 main methods.
def add_new_child(self, parent_id, child_id, child_option_title, child_description):
parent_node = self.current_node.find(parent_id)
new_child_node = Node(
id = child_id,
option_title = child_option_title,
description= child_description
)
parent_node.add_child(new_child_node)
def get_current_children(self):
return self.current_node.children
def set_current_node(self,chosen_node):
self.current_node = chosen_node
def is_finished(self):
return len(self.current_node.children) == 0
add_new_child()
: adds a new child node to a parent nodeget_current_children()
:returns a list of the children of the current nodeset_current_node(chosen_node)
: sets thecurrent_node
to thechosen_node
is_finished()
: returns True or False based on if the current node has children
[3] Building your first story #
๐
Let’s start taking a look at story_setup.py
As you can see, the current story has only has 4 unique Node()
objects and it call .add_new_child()
to build the story.
๐ป
Try to play through the short story in by running: python game.py
$ python game.py
==================================================
Title: Lunch.
It's lunch time.
Where will you go?
==================================================
[what will you do?]
โฏ Walk to cyberport.
Elevator down to A Block Cafeteria.
๐ง Hmmmmm…. it works, but it the story does not continue with your choice.
[Finish game.py] #
๐ป
Finish game.py
so it properly plays through the story. It should:
- loop through the given chosen options
- display the description of each chosen node
- end when there are no more options for the chosen node
๐ง Consider…:
- What methods exist in the
View()
andStory()
that you could use? - how do you loop until a condition is met?
๐พ Be sure to play test the game to ensure it works as expected: python game.py
[Continue the Story] #
Now that you’ve gotten a working game.py
, let’s build out the story.
Come up with your own options to continue the story! We’ll share out at the end of class.
๐ป
“Continue the story, add at least 3 unique Node()
objects. Some ideas…
- add additional lunch options in ISF A Block Cafeteria
- add lunch options in Fusion
- add other locations to purchase lunch in Cyberport
๐พ Be sure to play test the game to ensure your story additions work as expected: python game.py
[5] Deliverables #
โกโจ
Once you’ve successfully completed the game be sure to fill out this Google form.
๐ป Push your work to Github:
- git status
- git add -A
- git status
- git commit -m “describe your drawing and your process here”
be sure to customize this message, do not copy and paste this line
- git push
[6] Extension: #
For your project, you will need to build out one additional feature to this Story
framework. Here are a few suggested features:
- looping stories with the ability to set an Node as a current Node’s child
- e.g. player can go back to a previous area
- a
Player
class with unique properties- e.g. hunger, money, health
- variable messaging in the story
- e.g.
"you have visited this store 5 times"
- e.g.
- a special
Node
child-class that gives items or status effects- e.g. a locked door where you need to first collect the key in a certain room.
๐ป Use this time to experiment with of these features! If you have your own ideas, feel free to experiment with that!